수업 시간때는 제대로 이해하지 못하고 넘어간 내용인데
이번에 간단한 콘솔앱을 만드는 프로젝트를 하면서 짝꿍팀원이 커스텀 예외 처리를 한 것을 보고 너무너무 멋있어서👍 나도 다음에는 사용할 수 있는 사람이 되고자 개념을 정리하고 간단하게 만들어보았다
- 컴파일러가 체크하는 일반 예외로 선언할 경우, Exception을 상속받아서 구현
- 컴파일러가 체크하지 않는 실행 예외로 선언할 경우, RuntimeException을 상속받아서 구현
- 사용자 정의 예외 클래스 작성 시 생성자는 두 개 선언하는 것이 일반적
- 매개 변수가 없는 기본 생성자
- 예외 발생 원인(예외 메세지)을 전달하기 위해 String 타입의 매개변수를 갖는 생성자
- 예외 메세지의 용도는 catch{} 블록의 예외처리 코드에서 이용하기 위한 것
간단하게 해본 예제
- 생년월일 형식은 yyyy-mm-dd 으로 함
- 생년월일을 입력받고 형식에 맞으면 "정상적으로 입력되었습니다." 메세지 출력
- 생년월일을 구분하는 기호가 '-' 가 아니면 "입력 형식에 맞게 '-'로 구분해주세요" 메세지 출력
- 1000<=year<=현재year / 01<=month<=12 / 01<=day<=31 에 맞지 않는 날짜면 "입력 형식을 정확히 지켜주세요" 메세지 출력
- Date 객체를 통해 현재 년,월,일을 저장 후 입력한 생년월일이 현재 날짜보다 크다면 "현재 날짜보다 큽니다" 메세지 출력
- 정상적으로 입력한 경우
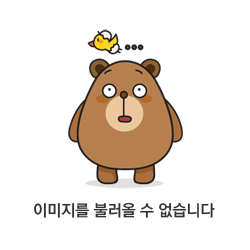
- 정상적으로 입력하지 않은 경우
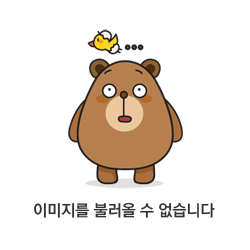
→ catch 안에 System.out.println(e.getMessage());
getMessage()
: String 타입의 매개변수 메세지를 갖는 생성자를 이용했다면 자동적으로 예외 객체 내부에 메세지를 저장하게 되는데 이 메세지를 리턴한다
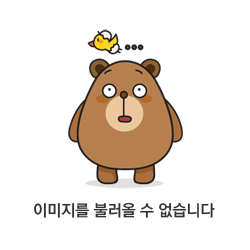
→ catch 안에 e.printStackTrace();
printStackTrace()
: 예외 발생 코드를 추적해서 어떤 예외가 어디에서 발생했는지 상세하게 출력해준다
try-catch문을 while(true)로 감싸주어서 정상적으로 입력했을 경우 break 로 종료 / 예외처리된 경우 continue 로 계속 입력할 수 있게 해주었다!
완성 코드,,,,인데 이게 맞나? 싶은 코드
Main.java
package practice2;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Date today = new Date();
//SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd");
SimpleDateFormat yearFormat = new SimpleDateFormat("yyyy");
SimpleDateFormat monthFormat = new SimpleDateFormat("MM");
SimpleDateFormat dayFormat = new SimpleDateFormat("dd");
int year = Integer.parseInt(yearFormat.format(today));
int month = Integer.parseInt(monthFormat.format(today));
int day = Integer.parseInt(dayFormat.format(today));
System.out.println("**생년월일을 입력하세요 (yyyy-mm-dd)**");
while(true) {
try {
String dateOfBirth = sc.next();
String[] dateOfBirthArray = dateOfBirth.split("-");
if(dateOfBirth.charAt(4) != '-' || dateOfBirth.charAt(7) != '-') {
throw new FormatException("입력 형식에 맞게 '-'로 구분해주세요");
} else if(Integer.parseInt(dateOfBirthArray[0]) < 1000 || Integer.parseInt(dateOfBirthArray[0]) > year
|| Integer.parseInt(dateOfBirthArray[1]) < 01 || Integer.parseInt(dateOfBirthArray[1]) > 12
|| Integer.parseInt(dateOfBirthArray[2]) < 01 || Integer.parseInt(dateOfBirthArray[2]) > 31) {
throw new FormatException("입력 형식을 정확히 지켜주세요");
} else if(Integer.parseInt(dateOfBirthArray[0]) == year) {
if(Integer.parseInt(dateOfBirthArray[1]) > month || Integer.parseInt(dateOfBirthArray[2]) > day) {
throw new FormatException("현재 날짜보다 큽니다");
}
}
break;
} catch (Exception e) {
System.out.println(e.getMessage());
//e.printStackTrace();
continue;
}
}
System.out.println("정상적으로 등록되었습니다.");
}
}
이렇게 길게 if문을 넣어도되는게 맞나? ,,🤔
FormatException.java
package practice2;
public class FormatException1 extends RuntimeException {
FormatException1() {
}
FormatException1(String msg) {
super(msg);
}
}
커스텀 예외 개념과 작성하는법을 익힐 수 있었다 다음 프로젝트때는 나도 꼭 사용해봐야지!!!
'혼자 > Java' 카테고리의 다른 글
[Java] next(), nextLine() 차이 (1) | 2024.01.04 |
---|---|
[Java] 객체 지향 문제 연습 (1) | 2024.01.03 |